Android EditText is a subclass of TextView. EditText is a standard entry widget in android apps. It is an overlay over TextView that configures itself to be editable
The EditText is the standard text entry widget in Android apps. If the user needs to enter text into an app, this is the primary way for them to do that. EditText
Edit Text example
activity_main.xml
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/rl"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="10dp"
tools:context=".MainActivity"
android:background="#f3f1eb"
>
<EditText
android:id="@+id/et"
android:layout_width="250dp"
android:layout_height="wrap_content"
android:padding="10dp"
android:layout_marginBottom="10dp"
android:hint="Multiline EditText by XML layout"
android:fontFamily="sans-serif-condensed"
android:background="#d3d7b6"
android:minLines="2"
android:maxLines="3"
android:scrollbars="vertical"
android:inputType="textMultiLine"
/>
<EditText
android:id="@+id/et2"
android:layout_width="250dp"
android:layout_height="wrap_content"
android:padding="10dp"
android:fontFamily="sans-serif-condensed"
android:hint="Multiline EditText programmatically"
android:background="#d4d7a5"
android:layout_below="@+id/et"
/>
</RelativeLayout>
MainActivity.java
package com.jigopost.edittext;
import android.os.Bundle;
import android.app.Activity;
import android.widget.EditText;
import android.widget.Scroller;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Get the widgets reference from XML layout
final EditText et = (EditText) findViewById(R.id.et);
final EditText et2 = (EditText) findViewById(R.id.et2);
/*
This will provide Scroll option for EditText,
but it will not show the scroll bar on EditText
*/
et2.setScroller(new Scroller(getApplicationContext()));
et2.setVerticalScrollBarEnabled(true);
/*
setMinLines (int minlines)
Makes the TextView at least this many lines tall.
Setting this value overrides any other (minimum) height
setting. A single line TextView will set this value to 1.
*/
// Set the minimum lines to display on EditText
et2.setMinLines(2);
/*
setMaxLines (int maxlines)
Makes the TextView at most this many lines tall.
Setting this value overrides any other (maximum) height setting.
*/
// Set the maximum lines to display on EditText
et2.setMaxLines(2);
}
}
Output
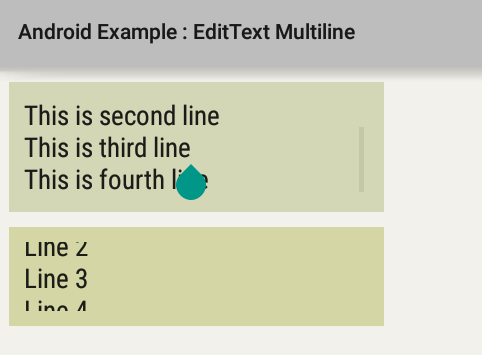
Next Tutorial