In this android programming source code example, we are going to change the border color of a Chip in Android.
Example of chip border color in kotli
MainActivity.kt
package com.jigopost.boardercolor
import android.content.Context
import android.content.res.ColorStateList
import android.graphics.Color
import android.os.Bundle
import android.util.TypedValue
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// set chip border color programmatically
chipYellow.chipStrokeColor = ColorStateList.valueOf(
Color.parseColor("#E30022")
)
// set chip border width in pixels programmatically
chipYellow.chipStrokeWidth = 3.dpToPixels(this)
}
}
// extension function to convert dp to equivalent pixels
fun Int.dpToPixels(context: Context):Float = TypedValue.applyDimension(
TypedValue.COMPLEX_UNIT_DIP, this.toFloat(), context.resources.displayMetrics
)
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#FEFEFA"
tools:context=".MainActivity">
<com.google.android.material.chip.ChipGroup
android:id="@+id/chipGroup"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="32dp"
android:layout_marginEnd="8dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<com.google.android.material.chip.Chip
android:id="@+id/chipCharcoal"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.MaterialComponents.Chip.Filter"
app:chipStrokeColor="#E30022"
app:chipStrokeWidth="3dp"
android:text="Charcoal" />
<com.google.android.material.chip.Chip
android:id="@+id/chipYellow"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:text="Yellow" />
</com.google.android.material.chip.ChipGroup>
</androidx.constraintlayout.widget.ConstraintLayout>
Output
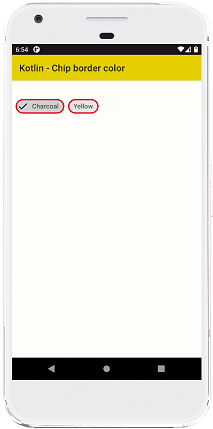