Android Volley JsonArrayRequest to Post ArrayList data to the server.Store Data in Locle recyclerView list and send to the server. JsonArrayRequest — A request for retrieving a JSONArray response body at a given URL
Example of volley json arrayRequest in kotlin
MainActivity.kt
package com.jigopost.volley
import android.os.Bundle
import android.text.method.ScrollingMovementMethod
import android.view.View
import androidx.appcompat.app.AppCompatActivity
import com.android.volley.Request
import com.android.volley.toolbox.JsonArrayRequest
import kotlinx.android.synthetic.main.activity_main.*
import org.json.JSONException
import org.json.JSONObject
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// make text view content scrollable
textView.movementMethod = ScrollingMovementMethod()
// url to get json array
val url = "https://pastebin.com/raw/Em972E5s"
// get json array from url using volley network library
button.setOnClickListener {
progressBar.visibility = View.VISIBLE
// request json array response from the provided url
val request = JsonArrayRequest(
Request.Method.GET, // method
url, // url
null, // json request
{response -> // response listener
try {
textView.text = ""
// loop through the array elements
for (i in 0 until response.length()){
// get current json object as student instance
val student: JSONObject = response.getJSONObject(i)
// get the current student (json object) data
val firstName: String = student.getString("firstname")
val lastName: String = student.getString("lastname")
val age: Int = student.getInt("age")
// display the formatted json data in text view
textView.append("$firstName $lastName\nAge : $age\n\n")
}
}catch (e: JSONException){
textView.text = e.message
}
progressBar.visibility = View.INVISIBLE
},
{error -> // error listener
textView.text = error.message
progressBar.visibility = View.INVISIBLE
}
)
// add network request to volley queue
VolleySingleton.getInstance(applicationContext)
.addToRequestQueue(request)
}
}
}
VolleySingleton.kt
package com.jigopost.volley
import android.content.Context
import android.graphics.Bitmap
import android.util.LruCache
import com.android.volley.Request
import com.android.volley.RequestQueue
import com.android.volley.toolbox.ImageLoader
import com.android.volley.toolbox.Volley
class VolleySingleton constructor(context: Context) {
companion object {
@Volatile
private var INSTANCE: VolleySingleton? = null
fun getInstance(context: Context) =
INSTANCE ?: synchronized(this) {
INSTANCE ?: VolleySingleton(context).also {
INSTANCE = it
}
}
}
val imageLoader: ImageLoader by lazy {
ImageLoader(requestQueue,
object : ImageLoader.ImageCache {
private val cache = LruCache<String, Bitmap>(20)
override fun getBitmap(url: String): Bitmap {
return cache.get(url)
}
override fun putBitmap(url: String, bitmap: Bitmap) {
cache.put(url, bitmap)
}
})
}
private val requestQueue: RequestQueue by lazy {
// applicationContext is key, it keeps you from leaking the
// Activity or BroadcastReceiver if someone passes one in.
Volley.newRequestQueue(context.applicationContext)
}
fun <T> addToRequestQueue(req: Request<T>) {
requestQueue.add(req)
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#EDEAE0"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:text="Get Json Array"
android:textAllCaps="false"
android:backgroundTint="#592720"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ProgressBar
android:id="@+id/progressBar"
style="?android:attr/progressBarStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:visibility="invisible"
app:layout_constraintBottom_toBottomOf="@+id/button"
app:layout_constraintStart_toEndOf="@+id/button"
app:layout_constraintTop_toTopOf="@+id/button" />
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="12dp"
android:layout_marginEnd="8dp"
android:fontFamily="sans-serif-condensed-medium"
android:padding="8dp"
android:textColor="#4F42B5"
android:textSize="22sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button"
tools:text="TextView" />
</androidx.constraintlayout.widget.ConstraintLayout>
add this line into app.gradle [dependencies]
// volley network library
implementation 'com.android.volley:volley:1.1.1'
Out Put
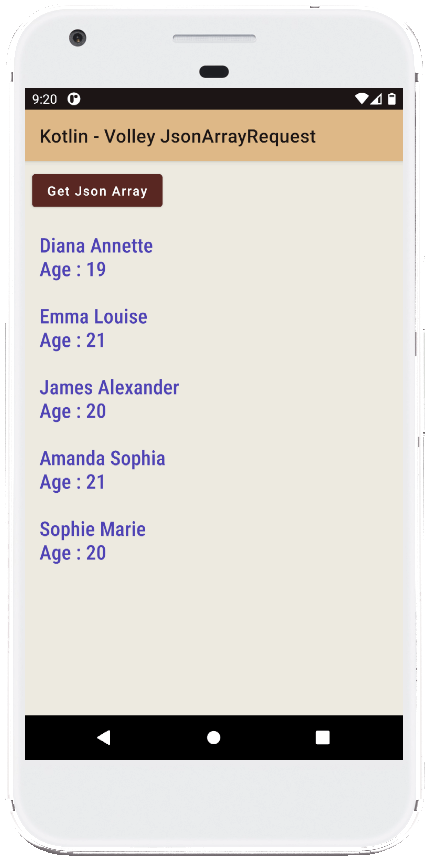