In this tutorial we will create a MultipleChoice AlertDialog using Android Studio. We will show list of colors to show in dialog. We will create a Button to show that AlertDialog, and a TextView to show the selected items…
Step 1: Create a new project OR Open your project
Step 2: Code
activity_main.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="20dp"
android:orientation="vertical"
tools:context=".MainActivity">
<Button
android:id="@+id/showsnackbarbtn"
android:text="Show Alert Dialog"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/txtView"
android:text="Preferred colors"
android:textSize="20sp"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
MainActivity.kt
package com.jigopost.myapplication
import android.support.v7.app.AppCompatActivity
import android.os.Bundle
import android.support.v7.app.AlertDialog
import android.widget.TextView
import android.widget.Toast
import kotlinx.android.synthetic.main.activity_main.*
import java.util.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val mSlctdTxtTv = findViewById<TextView>(R.id.txtView)
showsnackbarbtn.setOnClickListener {
val builder = AlertDialog.Builder(this@MainActivity)
// String array for alert dialog multi choice items
val colorsArray = arrayOf("Black", "Orange", "Green", "Yellow", "White", "Purple")
// Boolean array for initial selected items
val checkedColorsArray = booleanArrayOf(true, // Black checked
false, // Orange
false, // Green
true, // Yellow checked
false, // White
false //Purple
)
// Convert the color array to list
val colorsList = Arrays.asList(*colorsArray)
//setTitle
builder.setTitle("Select colors")
//set multichoice
builder.setMultiChoiceItems(colorsArray, checkedColorsArray) { dialog, which, isChecked ->
// Update the current focused item's checked status
checkedColorsArray[which] = isChecked
// Get the current focused item
val currentItem = colorsList[which]
// Notify the current action
Toast.makeText(applicationContext, currentItem + " " + isChecked, Toast.LENGTH_SHORT).show()
}
// Set the positive/yes button click listener
builder.setPositiveButton("OK") { dialog, which ->
// Do something when click positive button
mSlctdTxtTv.text = "Your preferred colors..... \n"
for (i in checkedColorsArray.indices) {
val checked = checkedColorsArray[i]
if (checked) {
mSlctdTxtTv.text = mSlctdTxtTv.text.toString() + colorsList[i] + "\n"
}
}
}
// Set the neutral/cancel button click listener
builder.setNeutralButton("Cancel") { dialog, which ->
// Do something when click the neutral button
}
val dialog = builder.create()
// Display the alert dialog on interface
dialog.show()
}
}
}
Step 3: Outpu
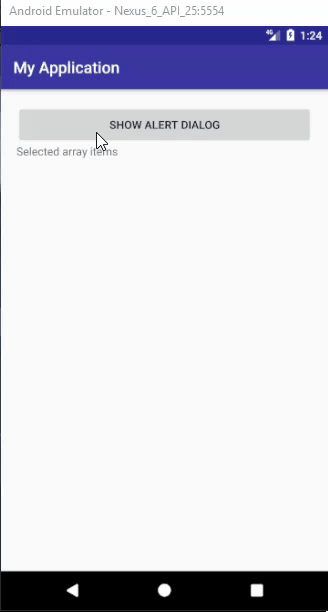