In this tutorial we will use Alert Dialog to alert the user whether you want to perform specific function or not. Alert Dialog will contain icon, title, message and two buttons “Yes”, “No”. We will show a alert dialog on button click.
Step 1: Create a new project OR Open your project
Step 2: Code
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="20dp"
tools:context=".MainActivity">
<Button
android:id="@+id/showsnackbarbtn"
android:text="Show Alert Dialog"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
MainActivity.kt
package com.jigopost.myapplication
import android.support.v7.app.AppCompatActivity
import android.os.Bundle
import android.support.v7.app.AlertDialog
import android.widget.Toast
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
showsnackbarbtn.setOnClickListener {
val mAlertDialog = AlertDialog.Builder(this@MainActivity)
mAlertDialog.setIcon(R.mipmap.ic_launcher_round) //set alertdialog icon
mAlertDialog.setTitle("Title!") //set alertdialog title
mAlertDialog.setMessage("Your message here") //set alertdialog message
mAlertDialog.setPositiveButton("Yes") { dialog, id ->
//perform some tasks here
Toast.makeText(this@MainActivity, "Yes", Toast.LENGTH_SHORT).show()
}
mAlertDialog.setNegativeButton("No") { dialog, id ->
//perform som tasks here
Toast.makeText(this@MainActivity, "No", Toast.LENGTH_SHORT).show()
}
mAlertDialog.show()
}
}
}
Step 3: Output
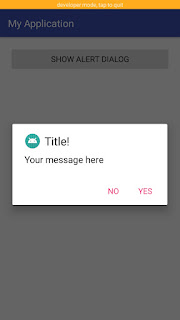