In this tutorial we will display all audio files from Internal Storage in the ListView. You can also display the audio files from External Storage using this tutorial.
Step 1: Create a new project OR Open your project
Step 2: Code
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:orientation="vertical"
tools:layout_editor_absoluteY="81dp">
<ListView
android:id="@+id/audioListView"
android:layout_width="match_parent"
android:layout_height="match_parent">
</ListView>
</LinearLayout>
MainActivity.java
package com.jigopost.myapplication;
import android.database.Cursor;
import android.os.Bundle;
import android.provider.MediaStore;
import android.support.v7.app.AppCompatActivity;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ListView mAudioListView = (ListView) findViewById(R.id.audioListView);
ArrayList<String> mAudioList = new ArrayList<>();
//detail of each audio
String[] mAudioDetailArray = { MediaStore.Audio.Media._ID, MediaStore.Audio.Media.DISPLAY_NAME};
//INTERNAL_CONTENT_URI to display audio from internal storage
//EXTERNAL_CONTENT_URI to display audio from external storage
Cursor mAudioCursor = getContentResolver().query(MediaStore.Audio.Media.INTERNAL_CONTENT_URI, mAudioDetailArray, null, null, null);
if(mAudioCursor != null){
if(mAudioCursor.moveToFirst()){
do{
int audioIndex = mAudioCursor.getColumnIndexOrThrow(MediaStore.Audio.Media.DISPLAY_NAME);
mAudioList.add(mAudioCursor.getString(audioIndex));
}while(mAudioCursor.moveToNext());
}
}
mAudioCursor.close();
ArrayAdapter<String> mAdapter = new ArrayAdapter<>(this,android.R.layout.simple_list_item_1,android.R.id.text1, mAudioList);
mAudioListView.setAdapter(mAdapter);
}
}
Step 3: Output
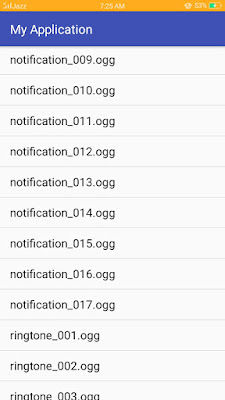