How to create a button and handle OnClickListener in Kotlin using Android Studio?
In this tutorial we will create a button and handle button click. We will display a Toast on the click of that button. However you can do your own functionality such as moving to some activity, opening fragment etc.
Step 1: Create a new Project or open new project
Step 2: Code
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Button"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
MainActivity.kt
package com.jigopost.myapplication
import android.support.v7.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
import android.widget.Toast
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val mBtn = findViewById<Button>(R.id.btn) as Button
mBtn.setOnClickListener {
Toast.makeText(this, "Button is clicked", Toast.LENGTH_LONG).show();
}
}
}
Step 3: Run Project
Output
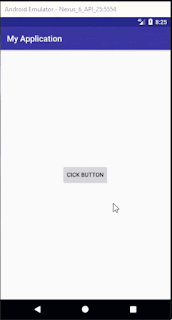