Chip checked color programmatically to set chip selected background color. change the background color of chip programmatically in Android. Example below
MainActivity.kt
package com.jigopost.chipcolor
import android.content.res.ColorStateList
import android.graphics.Color
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import androidx.core.view.children
import com.google.android.material.chip.Chip
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get chip group initially checked chips
handleSelection()
// set checked change listener for each chip on chip group
chipGroup.children.forEach {
val chip = it as Chip
chip.setOnCheckedChangeListener { buttonView, isChecked ->
handleSelection()
}
// set chip background color programmatically
// both for chip checked and unchecked states
chip.chipBackgroundColor = colorStates()
}
}
// function to get chip group checked chips
private fun handleSelection(){
textView.text = "Checked Chips : "
chipGroup.checkedChipIds.forEach{
val chip = findViewById<Chip>(it)
textView.append("\n${chip.text}")
}
}
}
// function to generate color state list for chip
fun colorStates(): ColorStateList{
val states = arrayOf(
intArrayOf(android.R.attr.state_checked),
intArrayOf(-android.R.attr.state_checked)
)
val colors = intArrayOf(
// chip checked color
Color.parseColor("#B8860B"),
// chip unchecked color
Color.parseColor("#BDB76B")
)
return ColorStateList(states, colors)
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#FEFEFA"
tools:context=".MainActivity">
<com.google.android.material.chip.ChipGroup
android:id="@+id/chipGroup"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="32dp"
android:layout_marginEnd="8dp"
app:singleSelection="false"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<com.google.android.material.chip.Chip
android:id="@+id/chipRed"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:text="Red" />
<com.google.android.material.chip.Chip
android:id="@+id/chipGreen"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:text="Green" />
<com.google.android.material.chip.Chip
android:id="@+id/chipYellow"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:text="Yellow" />
<com.google.android.material.chip.Chip
android:id="@+id/chipBlue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:text="Blue" />
<com.google.android.material.chip.Chip
android:id="@+id/chipBlack"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:text="Black" />
<com.google.android.material.chip.Chip
android:id="@+id/chipMagenta"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:text="Magenta" />
</com.google.android.material.chip.ChipGroup>
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:fontFamily="sans-serif-condensed-medium"
android:gravity="center"
android:padding="8dp"
android:textColor="#4F42B5"
android:textSize="22sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chipGroup"
tools:text="TextView" />
</androidx.constraintlayout.widget.ConstraintLayout>
Output
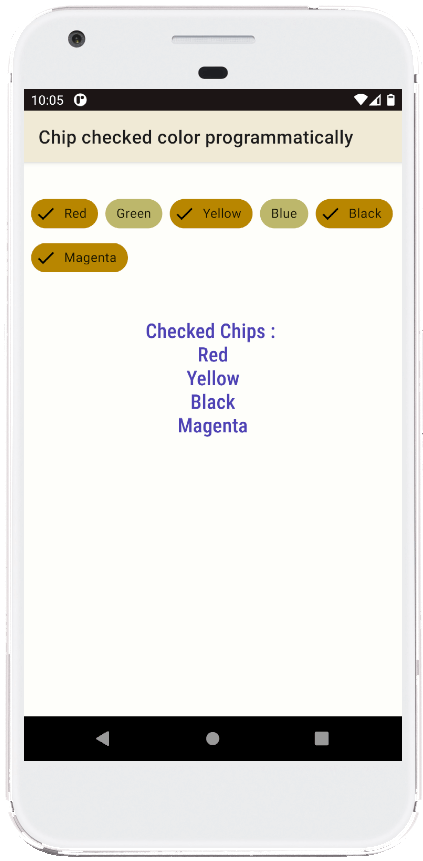